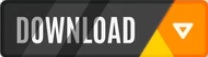
Next, we run the map method with a few arguments. The pool class creates by default one process per CPU core. Then, we create an instance of the Pool class, without specifying any attribute.
#Visual task psychopy example code code#
In this code snippet, we have a cube(x) function that simply takes an integer and returns its square root. map (cube, range ( 10 ,N ) ) print ( "Program finished!" ) sqrt (x ) if _name_ = "_main_" : with Pool ( ) as pool : This is our new example: from multiprocessing import Pool The Pool class allows you to create a pool of worker processes, and in the following example, we’ll look at how can we use it. What if we need to create multiple processes to handle more CPU-intensive tasks? Do we always need to start and wait explicitly for termination? The solution here is to use the Pool class. As you may guess, we can create more child processes by creating more instances in the Process class. In this example, we’ve created only one child process. The join() method waits for the process to terminate. At this point, the process is started.īefore we exit, we need to wait for the child process to finish its computations. Once we’ve created an instance to the Process class, we only need to start the process. args=(,), which is the array passed as argument to the target function.target=bubble_sort, meaning that our new process will run the bubble_sort function.Indeed, you can see that we’ve passed a few arguments: This class represents an activity that will be run in a separate process. The Process classįrom multiprocessing, we import the class Process. The crucial thing to know is that it’s a function that does some work. If you don’t know what it is, don’t worry, because it’s not that important. This function is a really naive implementation of the Bubble Sort sorting algorithm. In this snippet, we’ve defined a function called bubble_sort(array). P = Process (target =bubble_sort, args = (, ) ) Print ( "Array sorted: ", array ) if _name_ = '_main_' : Here’s the code we’ll use for this example: from multiprocessing import ProcessĬheck = False for i in range ( 0, len (array ) - 1 ) : if array > array : In this way, the parent can go on with its execution. We’re going to use the child process to execute a certain function. There’s only one parent process, which can have multiple children. In this example, we’ll have two processes: We’ll start with a very basic example and we’ll use it to illustrate the core aspects of Python multiprocessing. We’re finally ready to write some Python code! Getting Started with Python Multiprocessing The third advantage of multiprocessing is that it’s quite easy to implement, given that the task you’re trying to handle is suited for parallel programming. Since the comparison between multiprocessing and multithreading isn’t in the scope of this article, I encourage you to do some further reading on it. If you create a thread, it’s dangerous to kill it or even interrupt it as you would do with a normal process. Threads are not processes though, and this has its consequences. The second advantage looks at an alternative to multiprocessing, which is multithreading. Most processors are multi-core processors nowadays, and if you optimize your code you can save time by solving calculations in parallel. Since multiprocessing creates new processes, you can make much better use of the computational power of your CPU by dividing your tasks among the other cores.
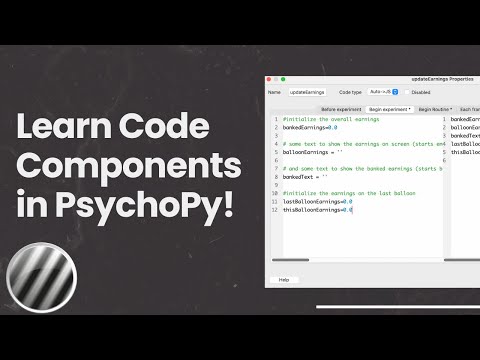
The first advantage is related to performance. more control over a child compared with threads.better usage of the CPU when dealing with high CPU-intensive tasks.I have tried several options including changing the dimensions in the excel files without decimals but the error message held.Here are a few benefits of multiprocessing: I attach the script and the excel files (which contains the rectangle dimensions and colours). ValueError: could not convert string to float: ‘ï»❳’ Size = float(row) # the first element in the row converted to a floating point number However, I get the following error: “File “C:\Users\USER\OneDrive\Área de Trabalho\psychopy\new_coding\untitled.py”, line 11, in I also have an excel file in csv formt which contains the dimensions (in first column) and color (second color) of the rectangel Enter image description hereI am trying to adapt an existing script to create a very simple experiment in psychopy in which the aim is that a rectangle with different colours and dimensions loops through the screen.
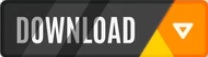